How to add Ant Design Components And Icons in a ReactJs application from CDN?
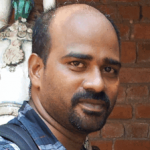
In general, everyone uses create-react-app for creating ReactJs applications. But, to create & deploy a simple ReactJS application with minimal dependency on the local file system, CDN based approach is the best.
In this article, let us see how to create a ReactJs application by referring ReactJs and Ant Design Components from CDN without installing them locally.
Creating ReactJs Application from CDN
It is not required to install NodeJs or NodeJs Packages in the local environment if we create a ReactJs application from CDN. Since we are trying to have the entire application in a single file, maintaining it would be very difficult.
Create an Html file with the below content and run it with the help of a local HTTP server.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello World</title>
<script src="https://unpkg.com/react@latest/umd/react.development.js" crossorigin="anonymous"></script>
<script src="https://unpkg.com/react-dom@latest/umd/react-dom.development.js"></script>
<script src="https://unpkg.com/babel-standalone@latest/babel.min.js" crossorigin="anonymous"></script>
</head>
<body>
<div id="root"></div>
<script type="text/babel">
let App = () => (<h1>Hello From ReactJs !!!</h1>)
ReactDOM.render(
<App />,
document.querySelector('#root'),
);
</script>
</body>
</html>
The above Html page will return an output similar to the one below.
Ant Design Components And Icons
Let us add references for Ant Design Components and Icons from CDN to the page created in the earlier step. Replace the content of the Html file with the below. This file contains CDN references to Ant Design Components and Ant Icons.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello World</title>
<script src="https://unpkg.com/react@latest/umd/react.development.js" crossorigin="anonymous"></script>
<script src="https://unpkg.com/react-dom@latest/umd/react-dom.development.js"></script>
<script src="https://unpkg.com/babel-standalone@latest/babel.min.js" crossorigin="anonymous"></script>
<!-- Ant Design Components and Icons -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/antd/4.18.5/antd.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/ant-design-icons/4.7.0/index.umd.min.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/antd/4.18.5/antd.min.css" />
</head>
<body>
<div id="root"></div>
<script type="text/babel">
const { Space, Input, Button, Layout } = antd;
const { Header, Content } = Layout;
const { Html5Outlined, EditOutlined } = icons;
function App() {
return (
<div>
<Layout style={{ width: '400px',padding:'20px', background: '#fff' }}>
<Header style={{ background: '#fff', textAlign: 'center', padding: 0 }}>
<h1>Hello From ReactJs <EditOutlined /></h1>
</Header>
<Content>
<Space direction="vertical">
<Input placeholder="Placeholder text" style={{ width: 200 }} />
<Button type="primary">Primary Button</Button>
<Html5Outlined style={{ fontSize: '32px', color: '#08c' }} />
</Space>
</Content>
</Layout>
</div>
);
}
ReactDOM.render(
<App />,
document.querySelector('#root'),
);
</script>
</body>
</html>
Here we are creating a Layout and placing a text box and a couple of Icons to showcase how Ant Components and Icons can integrate into an HTML page with the help of ReactJs.
This approach is fine for small applications that require minimal components or functionalities. You can choose this approach if the target environment/application allows only an HTML file or content to be embedded.
Leave a comment